La Maison d’Arrêt et de Correction de Ouagadougou (MACO) a abrité, ce vendredi 16 juin 2023, la restitution de formation humoristique dénommée « Sourire d’Espoir ». Il s’agit d’une initiative du groupe d’humoristes Génération 2000.
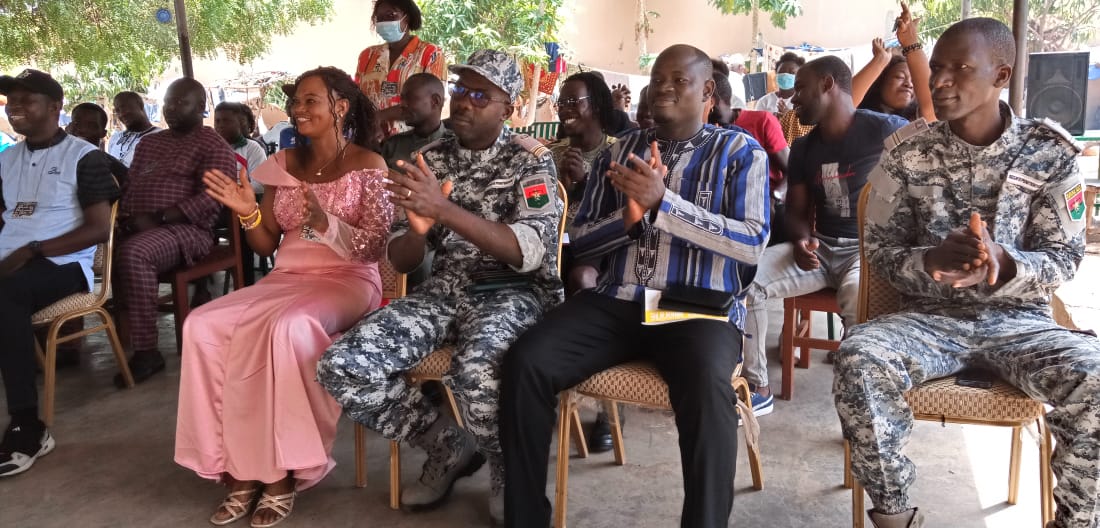
Pour la 3e année consécutive, le groupe d’humoristes Génération 2000 a réitéré sa coutume, celle d’initier des pensionnaires de la MACO aux outils humoristiques. Cette formation entre dans la dynamique de réinsertion prônée au profit des détenus de toutes les prisons du pays. Il s’agit en effet, à travers cette fenêtre ouverte, de non seulement leur donner un tout petit peu de sourire, mais aussi et surtout susciter de l’espoir en leur offrant l’opportunité de faire connaissances avec les différents outils de l’humour.
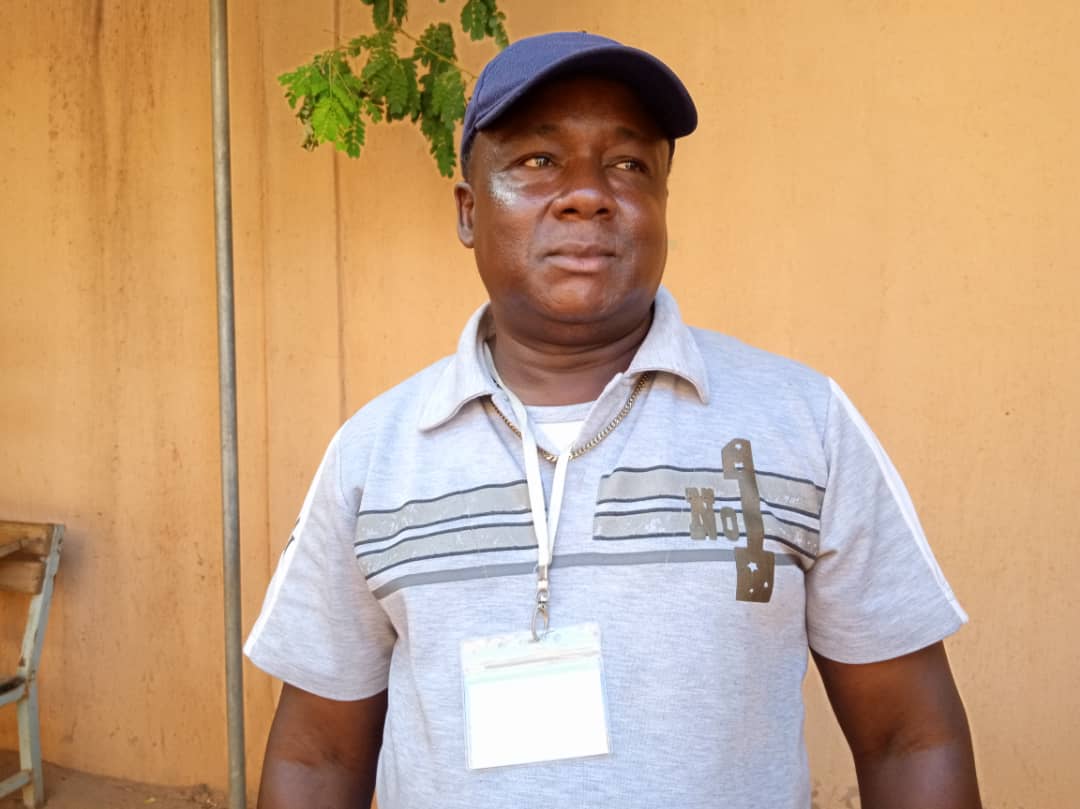
Ils étaient donc quatre, ces détenus, à bénéficier de cette formation. L’écriture de texte, la diction, la mise en scène, la gestuelle, tels ont été l’essentiel du contenu de cette formation. À en croire Neya Babou Michel alias Michou de Génération 2000, les détenus ont aussi droit aux opportunités. C’est ce qui justifie, selon lui, la mise en œuvre de ce projet. « Ceux que vous avez eu le plaisir de voir en prestations aujourd’hui, ont reçu des outils d’initiation à la 2e édition. Nous avons voulu continuer avec eux, afin de leur permettre de se perfectionner davantage. L’idée, c’est de pouvoir les suivre, tant que nous le pouvons, de sorte à ce qu’ils puissent en faire un métier à l’issue de leurs peines. D’ailleurs, seulement trois détenus ont presté par ce qu’il y a un qui a bénéficié d’une semi-liberté », a-t-il signifié.
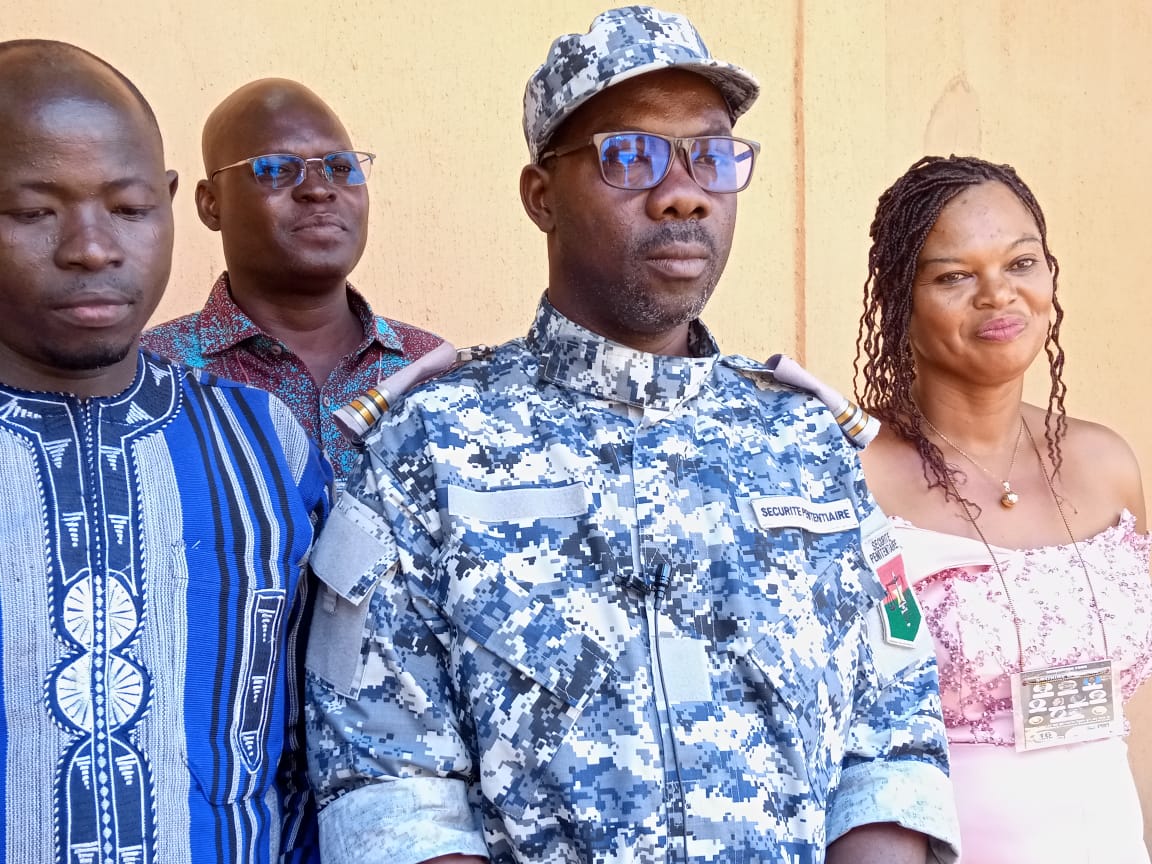
C’est donc devant leurs camarades détenus, humoristes confirmés et bien évidemment les premiers responsables de l’établissement pénitentiaire que ces probables futurs humoristes ont fait montre de leur talent. Frédéric Ouédraogo, Directeur de la MACO, a par ailleurs salué les initiateurs, qui depuis quelques années, font de leur mieux pour apporter de la joie dans les cœurs de ces détenus. « Les professionnels de l’humour qui étaient là aujourd’hui ont tous reconnu qu’il y a du talent à revendre. C’est pourquoi nous ne cesserons de réitérer notre gratitude à l’endroit du groupe Génération 2000 pour cette initiative. Nous espérons que d’autres gestes pareils suivront, pour le bonheur des détenus », a-t-il soutenu.
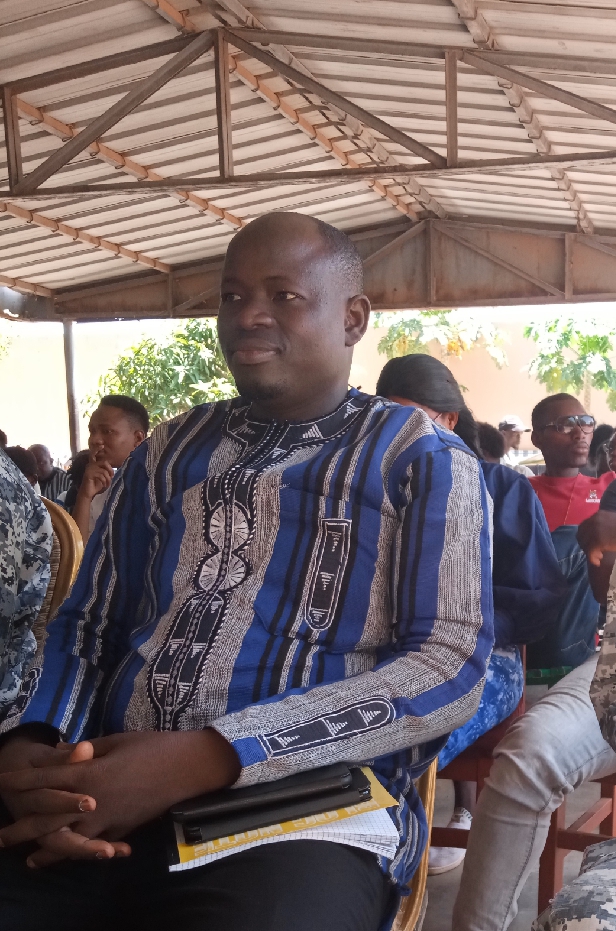
C’est une activité noble qui mérite amplement d’être encouragé. C’est du moins ce que nous confie le Juge d’Application des Peines (JAP) de Ouaga 1, Guelbéogo Lassané. Pour lui, « Sourire d’Espoir » permet non seulement aux détenus de se recréer mais aussi de s’essayer à des activités qui pourraient leur servir après avoir purger leurs peines. « Pour notre part, nous menons des réflexions pour voir comment les faire participer à des festivals. L’objectif est qu’ils puissent faire valoir leur talent dehors afin que les populations découvrent leur talent mais également se rendent à l’évidence que la prison n’est pas une fatalité », foi de monsieur Guelbéogo Lassané, le Juge d’Application des Peines (JAP) de Ouaga 1.
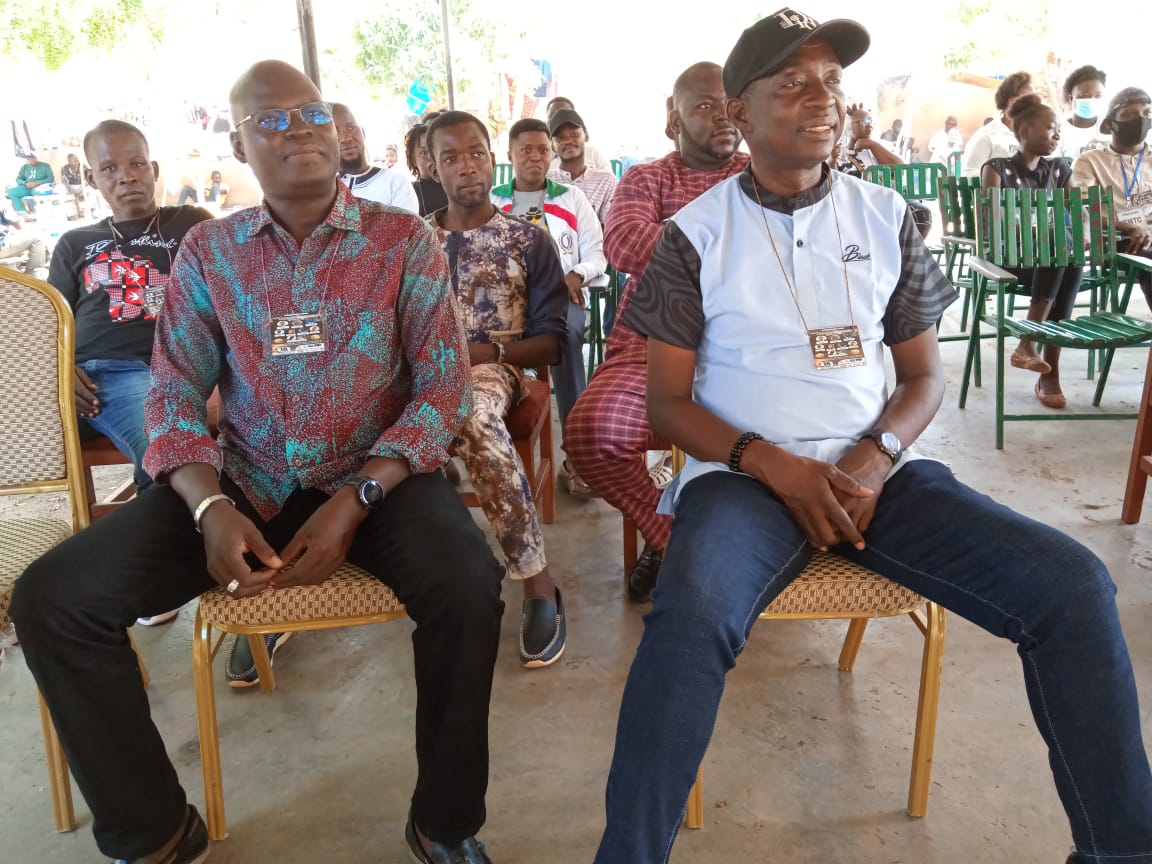
Une remise de cadeaux composés de vivres, est venue mettre fin à cette cérémonie de restitution. Les projecteurs sont d’ores et déjà tournés vers la 4e édition.
Boukari OUÉDRAOGO